The Overview
Below are two snippets of code that you need to make a phone call, record that phone call and download that recording. You can take these code snippets and run with it or you can follow along and we will break down some of the API functionality including authorisation, endpoints and POST/GET requests.
What we want to accomplish in this tutorial
- Set up a listening server that the 46elks API can post a response towards
- Make a phone call and record that phone call
- Get the data (recording/audiofile ) and download it to our local machine
What you will need for this tutorial
- Your 46elks API credentials. (Create an account if you dont already ahve one)
- A 46elks virtual phone number
- A local server - in this guide, we will use ngrok as a tunnel to our local server - visit ngrok.com to download or find out more information
Code Snippet #1 outgoing-call.py - Making a phone call and recording that phone call
import requests
auth = ('API_USERNAME','API_PASSWORD')
fields = {
'from': '+46766664341',
'to': '+46700036647',
'voice_start':
'{"record":"http://00165bb0.ngrok.io/recordings"}'
}
response = requests.post(
"https://api.46elks.com/a1/calls",
data=fields,
auth=auth
)
print(response.text)
Code Snippet # 2 capture-incoming-recording.py - Setting up a listening server to receive AND download audio file
from bottle import request, post, run
import requests
auth = ('API_USERNAME','API_PASSWORD')
@post('/recordings')
def recordings():
file_url = request.forms.get("wav")
download_file(file_url)
def download_file(url):
filename = url.split('/')[-1]
with requests.get(url, auth=auth, stream=True) as r:
r.raise_for_status()
with open(filename, 'wb') as f:
for chunk in r.iter_content(chunk_size=8192):
if chunk:
f.write(chunk)
return filename
run(host='0.0.0.0', port=5501)
Remember to initiate NGROK to forward traffic to your local server
You can do this with ./ngrok http 5501
. Find a quick-start guide for NGROK here.
Quicklinks for this tutorial
- Creating an outgoing phone call and recording the call
- Installing requests
- Authorising our request
- Sending the phone call to the 46elks /calls API endpoint
- The response from 46elks /calls API endpoint
- Setting the listening server and downloading the file
- Steps to test all components
Creating an outgoing phone call and recording the call
To begin, let’s create a folder called 46elks-recordings
.
Create a new file. Let’s call it outgoing-recording.py
because we want to make a phone call from our computer to a mobile
phone (outgoing) AND we want to record that call (recording).
Our file structure should now look like this:
46elks-recordings
outgoing-recording.py
The code
Copy this code and paste into outgoing-recording.py
.
We will go through the necessary parts of the code that you need to change.
For a full breakdown of each line by line you can find the extended guide here.
import requests
auth = ('API_USERNAME','API_PASSWORD')
fields = {
'from': '+46766664341',
'to': '+46700036647',
'voice_start':
'{"record":"http://00165bb0.ngrok.io/recordings"}'
}
response = requests.post(
"https://api.46elks.com/a1/calls",
data=fields,
auth=auth
)
print(response.text)
Installing requests
We are going to be using a python library called requests
and you might need to install it.
pip3 install requests
Authorising our request
Next, we need to authorise our request. To do this we can visit our account
dashboard and copy and paste the username and password. Here is a link to
your dashboard. Find out more about authentifcation here.
If you do not have an account you will need to create one.
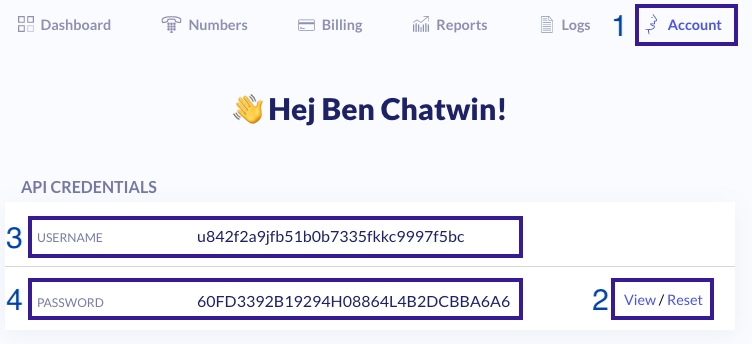
Replace your credentials in between the quotations ' '
auth = ('u842f2a9jfb51b0b7335fkkc9997f5bc',
'60FD3392B19294HO8864L4B2DCBBA6A6')
Sending the phone call to the 46elks /calls API endpoint
Now we can add the details of the request, so let’s talk about what the fields
mean.
'from'
= This is your 46elks virtual number which you can find in your dashboard.
The virtual phone number should be voice-enabled.
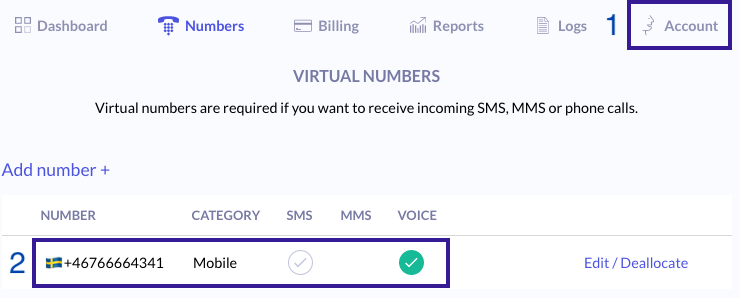
'to'
= This is the number that you want to call. We suggest calling your own mobile phone number during testing.
Copy and paste the URL from your server or NGROK tunnel that you set up and replace it in the code so it looks like this.
'voice_start':'{"record":"http://00165bb0.ngrok.io/recordings"}'
Be very careful not to mix up the ' ' and the " ". This is very important.
Time to test
Now run the python script.
$ python3 outgoing-recording.py
The response from 46elks /calls API endpoint
You should receive something similar to the response below AND your phone should ring!
{
"direction": "outgoing",
"from": "+46766664341",
"created": "2019-11-05T08:04:11.006257",
"to": "+46700036642",
"state": "ongoing",
"timeout": 60,
"owner": "u842f2a9jfb51b0b7335fkkc9997f5bc",
"id": "c30f413a464dfwerqr45492cb79f5cc84"
}
Answer your phone and you MUST say “46elks is fun and I cannot wait to show my team”.
But seriously, say whatever you want (be nice). The phone call will automatically stop recording after 3 seconds of silence or when you hang up.
46elks will record your call. You can check the latest logs in your dashboard to download the recording manually.
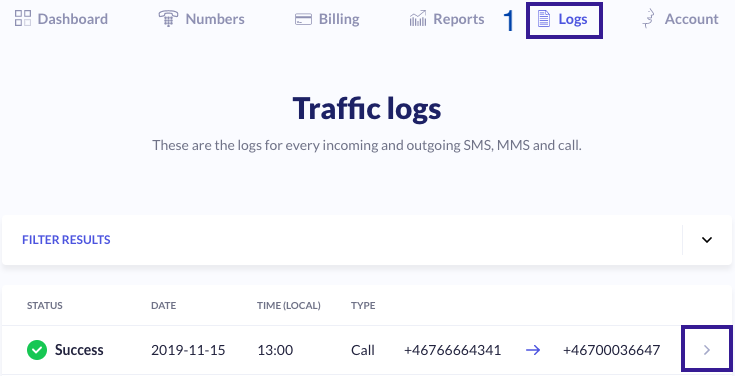
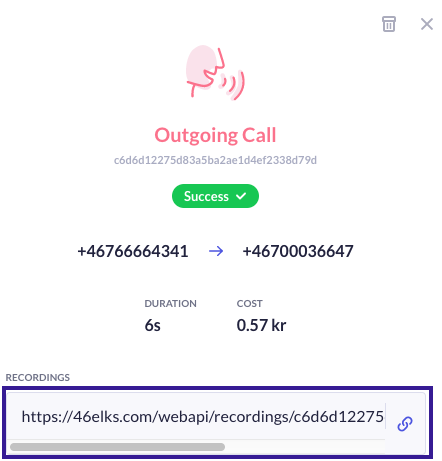
Simply click the chain icon and your download will begin. In the next step, we will learn how to download this file automatically to your computer.
Setting the listening server and downloading the file
Now that we have made the call, we need to set up a listening server that will download the file programmatically.
Create a new file. Let’s call it capture-incoming-recording.py
.
We want to listen for a request from the 46elks API that contains a URL to a recording.
We’ll visit that URL and automatically download the recording.
The code
Copy this code and paste into capture-incoming-recording.py
.
from bottle import request, post, run
import requests
auth = ('API_USERNAME','API_PASSWORD')
@post('/recordings')
def recordings():
file_url = request.forms.get("wav")
download_file(file_url)
def download_file(url):
filename = url.split('/')[-1]
with requests.get(url, auth=auth, stream=True) as r:
r.raise_for_status()
with open(filename, 'wb') as f:
for chunk in r.iter_content(chunk_size=8192):
if chunk:
f.write(chunk)
return filename
run(host='0.0.0.0', port=5501)
Installing bottle
We are going to be using a python library called bottle
which you might need to install.
pip3 install bottle
Authorising our request
Replace your credentials in between the quotations ' '
auth = ('u842f2a9jfb51b0b7335fkkc9997f5bc',
'60FD3392B19294HO8864L4B2DCBBA6A6')
Steps to test all components
Now we are ready to test our application. We can follow these steps:
- Initiate your server or NGROK tunnel (You probably did this already)
- Initiate the listening server
- Run capture-incoming-recording.py
python3 capture-incoming-recording.py
- Initiating the outgoing phone call
- Run outgoing-recording.py
python3 outgoing-recording.py
Initiating the listening server
$ python3 capture-incoming-recording.py
When you initiate capture-incoming-recording.py
you should see something similar to this:
Bottle v0.12.17 server starting up (using WSGIRefServer())...
Listening on http://0.0.0.0:5501/
Hit Ctrl-C to quit.
This means your server is active and listening. You need to leave this window
active which might require opening another tab/window to initiate outgoing-recording.py
in the next step.
Initiating the outgoing phone call
$ python3 outgoing-recording.py
You should receive something similar to the response below AND your phone should ring!
{
"direction": "outgoing",
"from": "+46766664341",
"created": "2019-11-05T08:04:11.006257",
"to": "+46700036642",
"state": "ongoing",
"timeout": 60,
"owner": "u842f2a9jfb51b0b7335fkkc9997f5bc",
"id": "c30f413a464dfwerqr45492cb79f5cc84"
}
Answer your phone. The phone call will automatically stop recording after 3 seconds of silence or when you hang up.
What success looks like
A new file should have been downloaded to your folder. Open up the audio file and try it out.
46elks-recordings
outgoing-recording.py
capture-incoming-recording.py
c30f413a464dfwerqr45492cb79f5cc84.wav
Need some more help or have some suggestions?
We would love to hear what you have to say. You can send us an email to help@46elks.com.