TL;DR version of the guide
You'll need to do the below steps and then copy the entire code is avaible below to be run on your server.
- Run a Flask enviroment on your local or live server
- A 46elks account
- A 46elks virtual phone number
- Remember to initiate NGROK to forward traffic to your local server
- The below code snippet
The full code
If you get stuck somewhere, these are the steps, so you can do some troubleshooting
- Setting up a Flask environment
- The code for app.py
- Configuring Flask endpoints
- Configuring your 46elks phone number
How to build an IVR application with Flask
What we want to accomplish in this tutorial
- Set up a listening server that the 46elks API can post a response towards
- Receive a phone call and play an audio file that contains a menu of two options:
- (1) Find out more about Voice
- (2) Find out more about SMS
- Based on whether the caller presses 1 or 2 play the associated audio file
What you will need for this tutorial
- A 46elks Account
- A 46elks virtual phone number
- A local server - in this guide, we will use ngrok as a tunnel to our local server.
Never used one before? Have a peak at this ngrok guide - Some basic understanding of how to use the command line
- There are some sound files hosted on 46elks.com which you are welcome to use for testing.
Setting up Flask
For a more comprehensive guide to setting up your first Flask project, we recommend following this tutorial Flask by Example – Project Setup.
If your still with us here, then follow these steps. This example uses python3.
- Create a new working directory/folder and enter it:
- Set up a virtual environment to use for our application:
- Next, we're going to create a file called flask-ivr-app.py and add it to your “flask-ivr” folder:
- Next, install flask:
The Python code
Open up your favorite editor and add the example code below to your file app.py
Configuring Flask endpoints
In the first section of code you will see this line of code:
The "next"
parameter is a url that 46elks will post towards with the details of which buttons are pressed. The "next"
parameter should be used anytime you want to continue the call. To put it a different way, anytime you offer callers an option of which you would like to know the outcome, you should provide a "next"
url.
In this case our Flask application will be running on an NGROK server and the location where we would like to receive information is at "/ivr"
where we have the next set of instructions.
Configuring your 46elks virtual phone number
We need to configure our 46elks virtual phone number so that our flask app is triggered whenever we receive a phone call. To do this head to your dashboard. In this example we are using NGROK, so we have added details of our NGROK server with /incomingCalls
on the end.
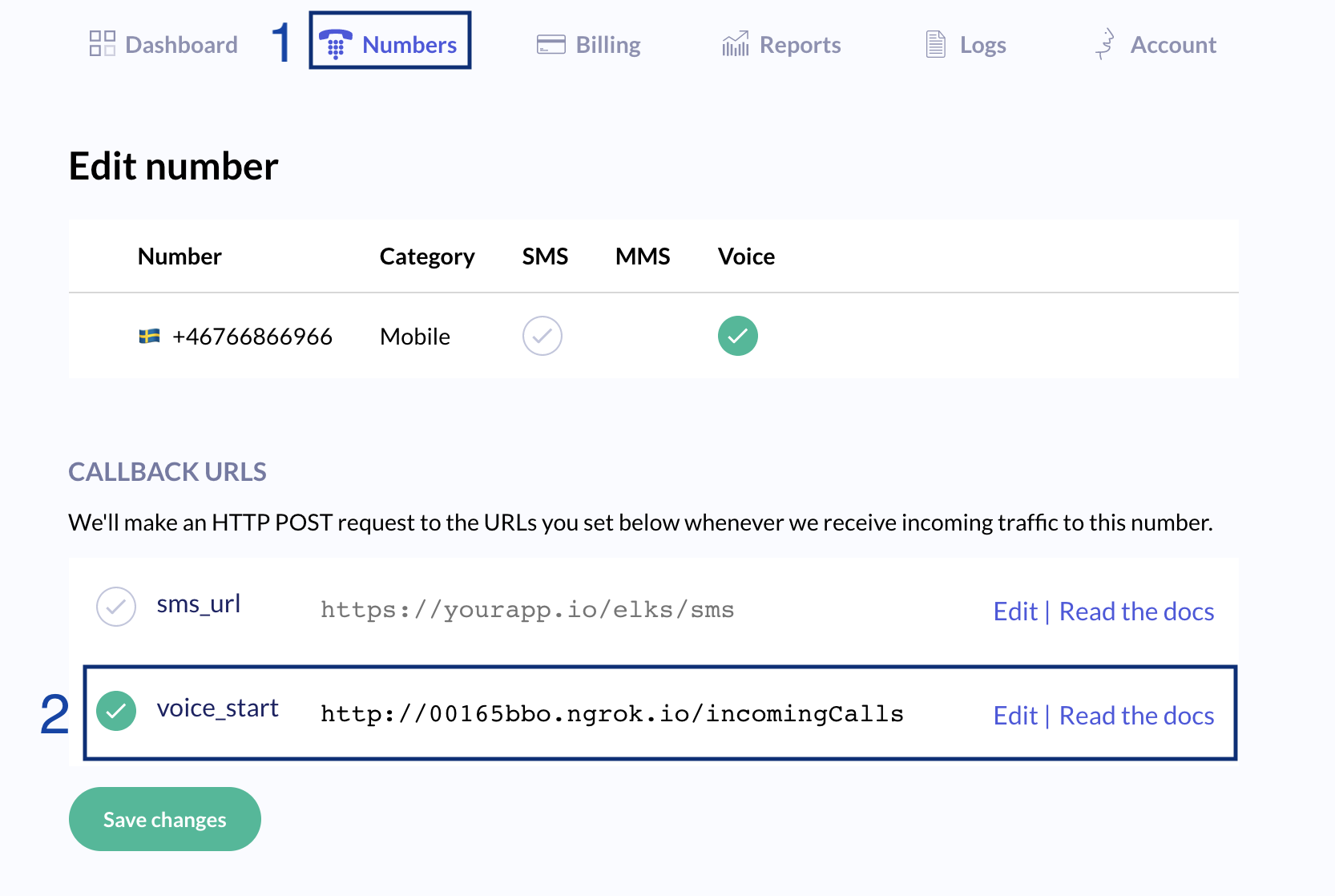
Now we are ready to test our app
You should now be able to test your application ☎️
- Initiate your server or NGROK tunnel if you have not already.
- Initiate
app.py
to start your flask application - Call your 46elks number to test
What success looks like
Below are two images. The first shows the terminal running the app.py server and the second is the NGROK server.
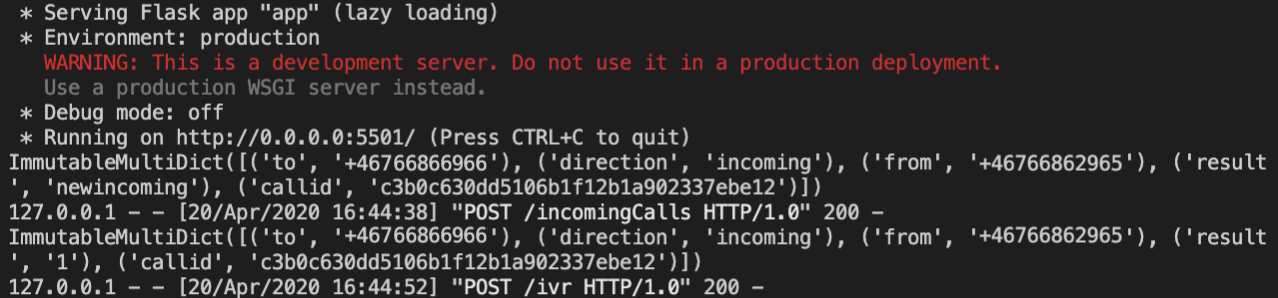
You can see in the image the different types of data that 46elks posts towards each Flask endpoint and how that data changes. In the example the caller selects the first option by pressing "1" and this is the result we obtain from the the API.
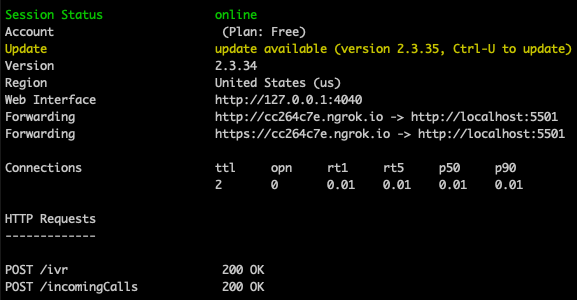
You can see in this image the two posts 46elks has made to the two Flask endpoints that we setup in our code example.
Need some more help or have some suggestions?
We would love to hear what you have to say. You can send us an email to help@46elks.com or call us at +46766861004.